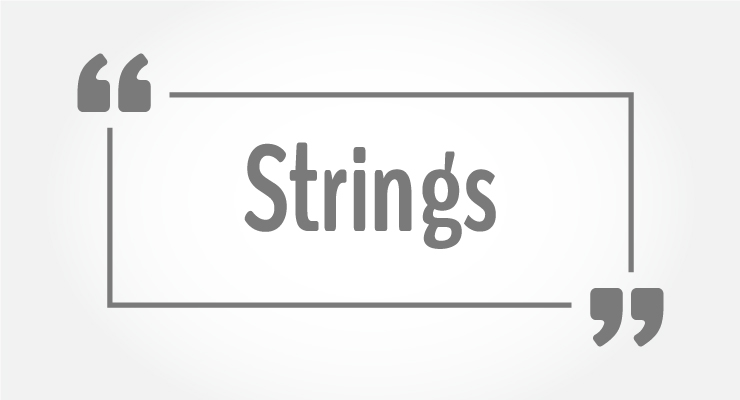
Introduction:-
“A string in an array of character.” Any group of characters (except double quotes) enclosed between double quotes is called a string constant. A string is a one-dimensional array of characters terminated by null ( \0).
For e.g. char s[10] = {‘s’,’h’,’i’,’v’,’a’,’m’,’\0’};
Each character in the array occupies one byte of memory and the last character is always a ‘\0’. This ‘\0’ is called a null. It defines the end of the string. Because of the presence of this character the various functions working on the string are able to know the end of the string. Thus it can be said that the input given to the string gets terminated at null ‘/0’. And the remaining array contains garbage values.
Note: the null ‘\0’ looks as if two characters are typed but the compiler treats it as a single character. It is an escape sequence. Also remember that ‘0’ is different from ‘\0’. ASCII of ‘0’ is 48 while ASCII of ‘\0’ is 0.
Character array initialization:-
1. char s[10];
2. char s[7] = {‘s’,’h’,’i’,’v’,’a’,’m’,’\0’};
3. char s[7] = {‘s’,’h’,’i’,’v’,’a’,’m’};
4. char s[7] = “shivam”;
5. char s[ ] = {‘s’,’h’,’i’,’v’,’a’,’m’,’\0’};
Let us observe a few important aspects of string:-
1. In the 3 declaration ‘\0’ character is missing i.e. a ‘\0’ is added automatically by the compiler at the end of the string.
2. The size of the string should be equal to the maximum number of characters in the string plus one.
3. The initialization of the string without mentioning of size is also permitted, as in the integer array. In such case the array size will be automatically based on the number of elements initialized.
String input:-
The common function used for input is scanf. This function can also be used to input the string using format specifier %s.
For e.g. scanf(“%s”,name);
While inputting the string the &(address of operator is not required). A problem with the scanf function is that it ends the input on the first white space it finds (a white space includes blank, tabs, carriage return, form feed, new line character). Therefore if the following line is typed as input.
Shivam Kumar
Then only the string “Shivam” will be taken as input into the variable name, because the blank space after word “Shivam” will end the string. Many times it is required to read the entire line of text, and it is not possible using the scanf. This can be achieved by using the getchar function. This function reads a single character at a time and store the character in the array. So we have to use this function repeatedly using a loop until the user types the null character or a new line character. Another function which can be used to input the string is called as gets( ). This function can also read the blank spaces and thus take a line as input in one go. and is used as:
gets(name);
String output:-
The commonly used output function printf( )can be used for string also using the format specifier %s to display the string on the screen. printf(“%s”,name); also the function puts( ) can be used. puts(name);
The precision of the string can also be specified%10.3 indicates that the first three characters are to be printed in the field width of 10 columns. 7 spaces will be printed before the string. But if the specification is (%-10.4) then the string will be printed as left justified and all the 7 spaces will printed towards the right.
1. Remember that if the field width is less than string length then string equal to string length will be printed.
printf(“%3.4”,name);
will print 4 characters not 3.
2. The integer value typed to the right side of the decimal point specifies the number of characters to be printed.
3. If the number of characters to be printed is specified as 0 then nothing is printed.
For Example:
char name[10]=”Shivam Kumar”;
printf(“%s”,name);
printf(“%.6s”,name);
printf(“%12.6s”,name);
printf(“%-12.6s”,name);
Output:
Shivam Kumar
Shivam
_ _ _ _ _ _Shivam
Shivam_ _ _ _ _ _