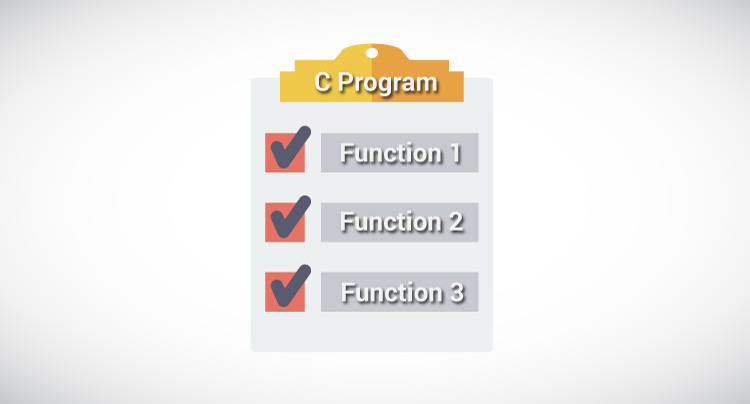
Introduction:-
Function is a small program which takes some input and gives us some output.
Function allows a large program to be broken down into a number of smaller self contained components, each of which has a definite purpose. It avoids rewriting the code over & over. Breaking down of logic into separate functions make the entire process of writing & debugging easier.
Function is a self contained block of statements that is used to perform some task. A function is assigned some work once and can be called upon for the task any number of times. Every C program uses some functions, the commonly used functions are printf, scanf, main,etc. Functions can be library functions or user defined functions.
Library functions are those functions which come along with the compiler and are present in the disk. The user defined functions are those which the programmer makes by himself to make his program easier to debug, trace.
printf ( ), scanf( ), exit( ), pow( ) are library functions. Every library function has a header file. There are a total of 15 header files in C. main( ) is a user defined function.
Every program must have a main function. The main function is used to mark the beginning of the execution. It is possible to code any program using only the main program but this leads to many problems. It becomes too large and complex thus difficult to trace, debug and test. But if the same program is broken into small modulus coded independently and then combined into a single unit then these problems can be solved easily. These modulus are called as functions.
Thus a function can be defined as a small program which takes some input and gives us some output.
Function to calculate the sum of two numbers:
int sum(int, int); /* Function Prototype or Declaration*/
main( )
{
int a,b,ans;
printf(“Enter two numbers: “);
scanf(“%d %d”,&a,&b);
ans= sum(a,b); /*Function Call*/
printf(“ sum is %d”, ans);
getch( );
}
int sum( int x, int y) /*Function Definition or Process*/
{
int z; z = x + y;
return(z);
}
Now let us see some of the features of this program:
1. The first statement is the declaration of the function which tells the compiler the name of the function and the data type of the argument passed.
2. The declaration is also called as prototype.
3. The declaration of a function is not necessary if the output type is an integer value. In some C compilers declaration is not required for all the function.
4. The function call is the way of using the function. A function is declared once, defined once but can be used a number of times in the same program. When the compiler encounters the function call, the control of the program is transferred to the function definition the function is then executed line by line and a value is returned at the end.
5. At the end of the main program is the definition, which can also be called as process.
6. The function definition does not terminate with a semicolon.
7. A function may or may not return any value. Thus the presence of the return statement is not necessary. When the return statement is encountered, the control is immediately passed back to the calling function.
8. While it is possible to pass any number of arguments to a function, the called statement returns only one value at a call. The return statement can be used as: return; or return(value); The first return without any value, it acts much as the closing of the braces of the function definition.
9. A function may have more than one return statement. It can be used as: if (a!=0) return(a); else return(1);
10. All functions by default return int. But if the function has to return a particular type of data the type specifiers can be used along with the function name. long int fact(n)
11. If function main( ) calls a function sum( ) then main( ) is the calling function and sum( ) is called function.