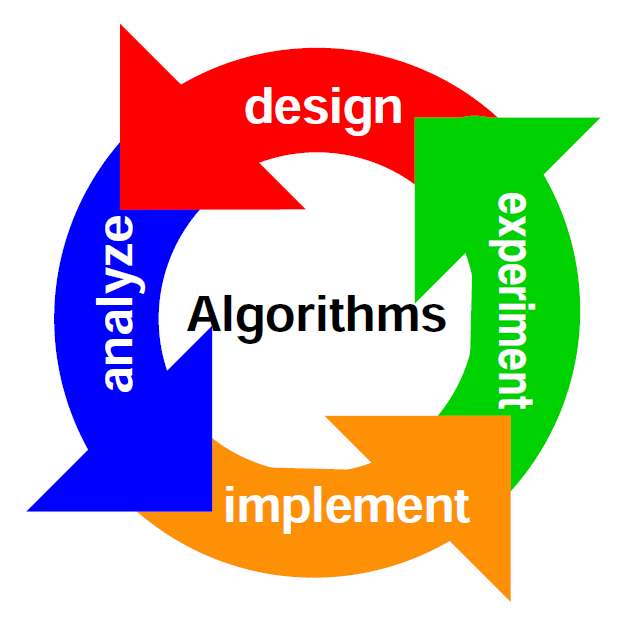
ALGORITHMS:-
Once a problem is been properly defined, a detailed, finite, step-by-step procedure for solving it must be developed. This procedure is known as algorithm. Algorithm can be written in ordinary language, or using procedures that lie somewhere between ordinary and programming languages.
Algorithm to add two numbers:
1. Read A, B.
2. Set SUM: = A+B.
3. Write SUM.
4. Exit.
PSEUDOCODE:-
Sometimes, it is desirable to translate an algorithm to an intermediate form, between that of a flowchart and the source code. Pseudocode is an English approximation of source code that follows the rules, style and format of a language but ignores most punctuation.
Example Of Pseudocode:
main ( )
{
integer a, b, sum;
read in a and b;
add a & b and set it to sum;
Write sum;
}
Structure of C program (Source Code):-
Let us discuss the structure of a C program using an example:
1. WAP to print the sum of two numbers.
#include<stdio.h> /* For print ( ) & scanf ( ) */
#include<conio.h> /* For clrscr( ) & getch( ) */
Main ( )
{
int a, b, sum;
clrscr( );
print (“enter two numbers”);
scanf(“%d%d”, &a,&b);
sum=a+b;
printf(“sum=%d”,sum);
getch( );
}
Important features of the above C program:
1. The instructions of a C program are typed in lowercase but the variables and user defined things can be written in uppercase.
2. The first line of this program is the comment. Every comment starts with slash and asterisk (/*) and ends with asterisk and slash (/*). A comment helps the programmer in explaining the logic of the program. It improves the readability of the program. These comments can be placed anywhere in the program. The compiler does not read these comments. Nesting of comments is not allowed. Comments are the explanation of a statement in the program to improve the readability of the program. Comments are not necessary; it is a good practice to begin a program with a comment indicating the purpose of the program, its author and the date on which the program was written. Any number of comments can be written at any place in the program example a comment can be written before the statement, after the statement or within the statement. A comment can be split over more than one line such a comment is called a multi-line comment.
3. The second and third lines of the program are called as header files (stdio.h and conio.h) which contain information that must be included in the program before compiling. # is a pre-processor directive or compiler directive. This statement directs the compiler to include header files in the program before compiling the program. We can also write <stdio.h> as “stdio.h”.
4. Every C program consists of one or more modules called functions. One of this functions. One of this function is called main ( ). The execution of every program begins with main function, which may call other functions. Whole program is written in this main function enclosed in curly brackets. Use of more than one main ( ) is illegal.
5. Declaration of the variables is done immediately after the opening braces of the program.
We cannot declare variables in the middle of a program.
6. Note that every statement in the main function ends with a semicolon (;).
7. Next is the printf( ) statement:- printf(“Enter two numbers”); printf is an output command which requests the user to enter a number. This message is known as “prompt message” and is printed on the output screen as Enter two numbers.
8. The values entered in the computer via the next statement scanf( ).
scanf(“%d%d”, &a,&b); scanf is an input command which takes some value from the user according to the given format specifier and stores it at the desired variable. The & is called as address of operator.
9. The next is the assignment statement:- sum=a+b; which adds the values in variable a and b and the assign it to variable sum.
10. The last printf( ) is used to show the calculated value for sum on the screen.
11. Finally the getch( ) function is used to show the output screen.
12. All the statements inside the main ( ) are slightly ahead than main ( ). This is called a indentation. This shows that all the statements are inside the main ( ) function.