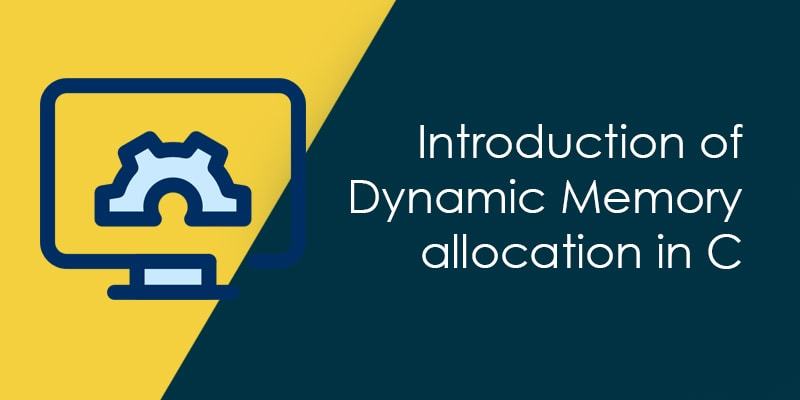
Memory Allocation In C Language
In C language the allocation of memory is basically of two types:
Static Memory Allocation
Dynamic Memory Allocation
The conventional way to use an array is by declaring the array with its size and since the size of array must be a constant so it leads to either wastage or shortage of memory because the array is allotted memory at the time of compilation of the program.
Static Memory The memory allocated at the time of compilation is called static memory. These problems can be overcome by the use of pointers.
Dynamic Memory Pointers can be used to allocate memory at the time of execution of the program, called dynamic memory. Thus dynamic memory can be described as the process of allocating memory at run time.
C does not inherently have this facility but they can be included in the program using the header file alloc.h
malloc( ) Allocates the requested number of bytes and returns the starting address to a pointer.
calloc( ) Allocates the requested number of bytes, initializes them with zero and returns the starting address to a pointer. It allocates the non continuous memory.
realloc( ) Reallocates the size by increasing or decreasing the no.of bytes and returns the starting address to a pointer.
free( ) Free previously allocated space.
Allocating a block of memory(malloc)
A block of memory may be allocated using the function malloc. The malloc function reserves a block of memory of specified size and returns a pointer of type void. This means we can assign the base address of the block to any type of pointer.
The general syntax of malloc is:
P= (cast type*) malloc(byte size);
Now let us see an e.g.
P=(int*)malloc (10 * sizeof(int));
Here the function malloc returns an integer type pointer p to an area of memory which
has the capacity to store 10 integer numbers. Similarly, the statement
P=(char*)malloc(10*sizeof(char));
Or
P=(char*)malloc(10); /*remember each character uses one byte*/
Allocates 10 bytes of space for the pointer p of character type.
This can be illustrated as:
Space to store 10 characters
Address of first byte
malloc can be used to allocate the space for complex data types such as structure:
str_p= (struct store *)malloc((sizeof(struct store));
where str_p is a pointer of type struct sore.
Note: malloc is used allocate the memory of contiguous bytes. The request can fail if memory
space is not sufficient to satisfy the request. If it fails, it returns a NULL.
Allocating multiple blocks (calloc)
Calloc is also a memory allocation function which is generally used to allocate memory for array and structure. malloc is used to allocate a single block storage space, calloc allocates multiple blocks of storage, each of same size and initializes them with zero.
The general syntax of calloc is:
P= (cast type*) calloc(n, array size);
Now let us see an e.g.of calloc
P =(int*)calloc (10 , sizeof(int));
The above statement allocates contiguous space for 10 blocks, each of size 2
bytes(since int requires two bytes). All the bytes are initialized to zero and a pointer gets the
starting address of the block allocated. Like malloc here also if the memory request cannot be
fulfilled a NULL is returned.
Altering the size of block
It is likely that we discover later, that the previously allocated memory is not sufficient and additional space would be required or the allocated memory is quite large than needed. In both these cases the size of the block has to be altered this can be achieved by using function realloc (reallocating memory).
The general syntax of realloc is:
P= realloc(P, new size);
Note: the new size can be larger or smaller than the previous one.
Now let us see an e.g. suppose the block for storing 10 integers was allocated using the malloc
P=(int*)malloc (10 * sizeof(int));
Now to alter the size so that 5 more numbers can be stored the statement would be:
P= realloc(P, 15*sizeof(int));
The function allocates a new memory space of size 15 to the pointer P and returns a
pointer to the first byte of the new block.
Also remember that the new memory block may or may not begin at the same place as
the old one. In such a case it creates a new memory space at some other place, copies all the old
data there and returns the block address to the pointer.
Releasing the used space In C
If the variables are declared at compile time they are destroyed according to their storage classes, but if the dynamic allocation is used it is the duty of the programmer to release the space if not in use. It is also necessary if the storage space is limited. We can release the memory space if the data at that space is not required by using the function free as: free(p);