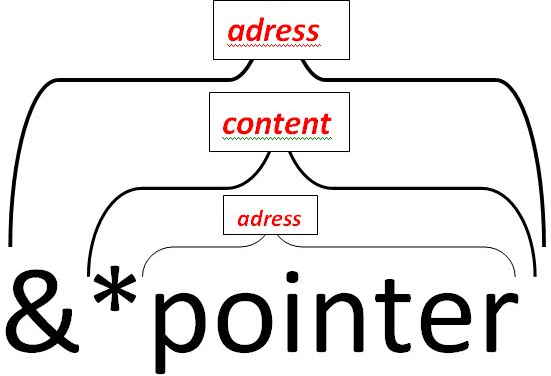
Pointers Expression:-
Like any other variables, pointer variable can be used in expressions.
In a program all the following operations are valid.
1. C allows us to add or subtract integers from pointers.
sum=sum +*p1;
2. Also short hand operators are allowed sum+=*p1; ans = *p1++;
This will cause the pointer p1 to point to the next value of its type the deference the value.
Let us use an e.g. to illustrate the point:
main( )
{
int a[5]={10,20,30,40,50},*p;
p=a /*address of array can be pointed into the pointer without the use of & operator*/
printf(“%d,”,*++p); /*dereferencing the next address*/
printf(“%d”,++*p); /*dereferencing the address and adding 1 to the element*/ }
Output:
20, 21
3. As well as to subtract one pointer from another
*p1–;
main( )
{
int a[5]={10,20,30,40,50},*p;
p=a;
printf(“%d,”,*++p); /*dereferencing the next address i.e. index 1*/
printf(“%d”,*p–); /*dereferencing the index 1 and then the postfix will affect*/
printf(“%d”,*p); /*dereferencing the pointer*/
}
Output:
20, 20, 10
4.If p1 and p2 are both pointers to the same array, then p2-p1 gives the number of elements between p1 and p2 *p3 = *p2 – *p1;
5.Two pointers can also be compared such as
p1>p2
p1= = p2
p1! = p2
These comparisons are useful only while using string or arrays.
6. Two pointers cannot be multiplied divided or added.
A null pointer is a special pointer value that is known not to point anywhere.
It means that no other valid pointer, to any other variable or array cell or anything else, will ever compare equal to a null pointer.
Another pointer variable is, by using any one of the standard header files, including <stdio.h>, <stdlib.h>, and <string.h>.
To initialize a pointer to a null pointer, you might use code like
#include <stdio.h>
int *p = Null;
and to test it for a null pointer before inspecting the value pointed to you might use code like
if(p != Null) printf(“%d \n”, *p);
It is also possible to refer to the null pointer by using a constant 0, and you will see some code that sets null pointers by simply doing
int *p = 0;
Furthermore, since the definition of “true” in C is a value that is not equal to 0, you will see code that tests for non-null pointers with abbreviated code like
if(p) printf(“%d\n”,*p);
This has the same meaning as our previous example;
if(p) is equivalent to if(p != 0) and to if(p!= NULL).