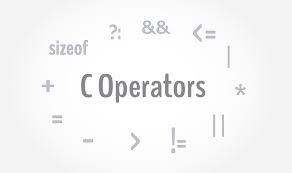
OPERATORS IN C
Operators: Operators can be used to perform the required computations on the values.
Types of Operators: There are three types of operators:
a) Unary Operator: Operators which work on one operand only.
b) Binary Operator: Operators which work on two operands.
c) Ternary Operator: Operators which work on three operands.
The Rule of Precedence: – Each operator in C has precedence associated with it. This precedence is used to determine how an expression involving more than one operator are evaluated. There are distinct levels of precedence and an operator may belong to one of the levels. The operators at the higher level of precedence are evaluated first.
The Rule of Associativity: – The operators of the same precedence are evaluated either from left to right or from right to left, depending on the level. This is known as the associativity property of an operator. There are 45 operators in C:
Classes of Operators: Operators are divided into mainly 9 classes:
1. Arithmetic operator
2. Unary operators
3. Relational operators
4. Assignment operators
5. Equality operators
6. Logical operators
7. Conditional operators
8. Bitwise operators
9. Comma operators
1) Arithmetic Operator: – These operators include +, -, *, /, % the + and – operators here are used to add and subtract two operands respectively. * is used to multiply while / is used to divide.
int i=2,j=3,k,l;
float a,b;
k=i/j*j;
l=j/i*i;
a=i/j*j;
b=j/i*i;
printf(%d, %d, %f, %f”,k,l,a,b);
Output: – 0, 2, 0.000000, 2.00000
% is called modulus operator, this operator is used to calculate remainder.
int a=5,b=3,c;
c= a%b;
printf(“%d”, c);
Output:- 2
Note: The modulus operator does not work on floating numbers.
2) Unary operators: – These operators include +,-,++,–, size of, (typecasting) the + and – in the unary operators are used to show the sign of the numbers. The ++operator is used to add 1 to the variable.
int a=5, b=4;
a++;
b–;
printf(“%d,%d” ,a,b);
Output: – 6, 3
If we use a++ or ++a both will increase the value of a by 1. a++ is called postfix while ++a is called prefix.
Postfix
int a=5,b;
b=a++;
printf(“%d, %d”,a,b);
Output:- 6,5
Prefix
int a=5,b;
b=++a;
printf(“%d, %d”,a,b);
Output:- 6,6
3) Relational Operator: – These operators include <, >, <=, >= .These operators are Boolean opearators (those operators which give answer in 1 or 0. Here 1 is considered true and 0 is false).
Ex1:
int x=10,a,b;
a= x>2;
b= x<2;
printf(“%d, %d”,a,b);
Output:- 1,0
Ex2:
int x=10,y=20,z=5,i;
i=x<y<z;
printf(“%d”,i);
a. 1
b. 0
c. Error
d. None of the above
Output:- a
4) Assignment operator: – These operators include =,+=,-=,*=,/=,%= . These operators are used to assign value to a variable.
a=5;
/* assigning 5 to variable a*/
a=5+2;
/* valid */
a=b;
/* valid */
5=a;
/* invalid */
a+b=5;
/* invalid */
The other operators of assignment are used for doing an arithmetic operation on a variable and then assigning the value to the same variable:
a+=5; is equivalent to a=a+5
a-=5; is equivalent to a=a-5
a*=5; is equivalent to a=a*5
a/=5; is equivalent to a=a/5
a%=5; is equivalent to a=a%5
5) Equality operator: – These operator includes = =,!= These two operators are used to check whether the given expression has the right and left sides equal or not. This operator is also a Boolean operator it also gives answer in 0 or 1.
int a,b,c=5;
a= =5;
b!=2;
printf(“%d, %d”,a,b);
Output:- 1,0
6) Logical Operator: – These operator includes &&, ||, ! These operators are also Boolean operators. && and || operator are used with two expressions. These expressions generally have either relational operators or equality operators in them.The Not operator is used to reverse the expression.
int a=10, b=5,c,d;
c= a>3 && b!=3;
d= (a= =10 ¦¦ b>20);
printf(“%d, %d”,c,d)
Output:- 1,1
Using the short circuit operator:
int a=5,b=4,c;
c= (b = =3 && a++);
printf(“%d, %d, %d”,a,b,c);
int a=10, b=5,c,d;
c= a>3 && b!=3;
d= (a= =10 ¦¦ b>20);
printf(“%d, %d”,c,d)
Output:- 1,1
Using the short circuit operator:
int a=5,b=4,c;
c= (b = =3 && a++);
printf(“%d, %d, %d”,a,b,c);
Output:- 5,4,0
Now let us try to understand this output, in the expression there are four types of operators, b=3 has equally operator. a++ is a unary operator. These two expressions are connected to each other with a logical AND. And at last value is assigned to variable c.
In the expression b = = 3 in a false expression thus would give 0. The next operator is a logical AND, this operator works on two different expressions (AND gives answer as 1 only if both the expressions are true) the first one being b = = 3 and the second one a++. The first expression for AND is false so it will not evaluate the next expression to speed up the execution of the program.
int i=3,j=2,k=0,m;
m = ++i && ++j || ++k;
printf(“%d %d %d %d”, i , j, k,m);
Output:-2 3 0 1
int i=3,j=2,k=0,m;
m = ++j && ++i || ++k;
printf(“%d %d %d %d”, i , j, k,m);
Output:-2 3 0 1
int i=3,j=2,k=0,m;
m = ++i || ++j && ++k;
printf(“%d %d %d %d”, i , j, k,m);
Output:-2 2 0 1
int i=3,j=2,k=0,m;
m = ++I && ++j && ++k;
printf(“%d %d %d %d”, i , j, k,m);
Output:-2 3 11
7) Conditional Operator: – This is the only ternary type of operator in C. It works on three operands. This class of operators is a set of two operators(? and 🙂 which work together. Let us understand the syntax of this operator first:
Condition ? true statement : false statement;
The above expression says if condition is true i.e. if it returns a non zero value, then the value returned will be true statement, otherwise the value returned will be false statement. The limitation of the conditional operators is that only one statement is allowed after ? or : .
Consider the following e.g.
int a=5,b=4,c;
a>b? max = a: max = b;
Output:- This will give an error ‘Lvalue required’. The error can be overcome by enclosing the statement in the : part within a pair of parenthesis. a>b? max = a: (max = b); In absence of parentheses the compiler believes that b is being assigned to the result of the expression to the left of second = . Hence it reports an error.
8) Bitwise operator: – Some programs require working on the bits(0 and 1) such as programs interacting with the hardware parts of the computer. Thus C contains several special operators which allow working on the bits. The bitwise operators are generally categorized into three types:
a) The one’s complement operator (~)
b) The logical bitwise operator. (&, |, ^)
c) The shift operators. (<<, >>)
In all three are 6 bitwise operators.
One’s complement operator: Also referred as the complementation operator. It is a unary operator that inverts the bits of the operand, i.e. all 0’s become 1 and all 1,s become 0’s. The operand for the operator must always be an integer value(int, short int, long int, unsigned, char).
Unsigned int a=5, b;
b = ~a;
printf(“%u”,b);
Output:-will be 65530.
Let us evaluate the output now: the binary equivalent of the number 5 will be 0000 0000 0000 0101.
As already said one’s complement converts all the 1s to 0s and all 0s to 1s. so the complement
will be 1111 1111 1111 1010 and converting the number to a decimal number will give
1*2 15 +1*2 14 +1*2 13 +1*2 12 +1*2 11 +1*2 10 +1*2 9 +1*2 8 +1*2 7 +1*2 6 +1*2 5 +1*2 4 +1*2 3 +1*2 2 +1*2 1 +0*2 32768+16384+8192+4096+2048+1024+512+256+128+64+32+16+8+0+2+0 and the final answer will be 65530.
32768+16384+8192+4096+2048+1024+512+256+128+64+32+16+8+0+2+0 and the final answer will be 65530.
The logical bitwise operators: – There are three logical bitwise operators bitwise AND(&), bitwise OR( |), bitwise OR( ^ ). Each of these operators requires two integer values to work. Each of the operands are individually converted into their respective binary numbers. The left most bit of the binary number is called the most significant bit or MSB. While the right most bit is called the least significant bit.
The shift operators: The two bitwise shift operators are left shift (<< ) and the right shift (>>). Each of these operators also requires two operands. The first integer operand that represents the bit pattern to be shifted. The second operand indicates the number of bits to be shifted.
int a,b;
a=20<<2;
b=20>>2;
printf(“%d %d”,a,b);
Output:- 80 , 5
The program will convert 20 to its binary equivalent i.e. 0000 0000 0001 0100 shifting 2 bits to the left would result the bits to be 0000 0000 0101 0000 and the integer number will be 80. If the bits of the integer number 20 are shifted 2 bits to the right side the binary number will be 0000 0000 0000 0101. Thus shifting the number to right by 1 reduces the number to half, while shifting the number to left by 1 bit doubles the number.
9) Comma operator: – , Operator works from left to right but returns the right most value. This operator is generally used in the for loop. The expressions separated by comma operator are solved from left to right. On using the comma operator the value and the type of right most operand is returned.
For e.g. in the assignment statement below:
I = (j = 1, k = 2, 3, 4);
The expression j = 1, k = 2, 3, 4 are evaluated first. Then the value of the expression is
returned as 4 and assigned to i.