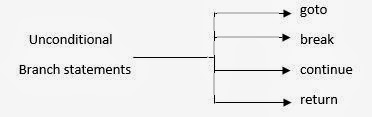
Jump statements
Loop performs a set of statements, till the condition becomes false. The number of times a loop is to be repeated is decided in advance and a condition is set. But sometimes it becomes necessary to skip certain part of the loop or terminate the loop if a particular condition is true then jump statements come to action:
C supports four jump statements:
i. break
ii. continue
iii. goto
iv. return
i) break
The break statement is used inside a loop, to directly come out of the loop. We have already used break in switch statement. It is used to skip the execution of the loop any further and transfer the control of the program to the statement following the loop.
Here loop counter condition from 1 to 10 and we will use break and continue when counter’s value is 7, let’s see what will be the output.
#include < stdio.h >
int
main()
{
int
cnt;
for
(cnt=1; cnt<=10; cnt++)
{
printf
(
"%d "
,cnt);
if
(cnt==7)
break
;
printf
(
"OK\n"
);
}
printf
(
"\nAfter the loop cnt= %d"
,cnt);
return
0;
}
ii) continue
Sometimes it is necessary to skip the execution of the statement when certain condition is true. continue does not terminate the loop but jumps directly to the next iteration. In for loop continue brings the control to the next increment or decrement but in while or do while loop continue causes the control of the loop to jump directly to the condition.
When cnt==7, break will transfer the program’s control just after the loop statement ( after the closing brace “}” ).
#include < stdio.h >
int
main()
{
int
cnt;
for
(cnt=1; cnt<=10; cnt++)
{
printf
(
"\n%d "
,cnt);
if
(cnt==7)
continue
;
printf
(
"OK"
);
}
printf
(
"\nAfter the loop cnt= %d"
,cnt);
return
0;
}
iii) goto
It is also used to jump from one part of the program to the other or exit from the deeply nested loop. But using goto is not a good practice because it makes the logic complicated and long programs unreadable.
*To print numbers from 1 to 10 using goto statement*/ #include < stdio.h > int main() { int number; number=1; repeat: printf ( "%d\n" ,number); number++; if (number<=10) goto repeat; return 0; } |
iv) return
Used in a function to return some value and to jump from called function definition to calling function definition.
// function with no return type void myFun( void ) { printf ( "Hello" ); return ; printf ( "Hi" ); } // will not print Hi // function with int return type – to return addition of two numbers int add( int a , int b) { int sum=0; sum=a+b; return sum; } // will transfer program’s control to calling function with value of sum. |
Library Function:-
exit( )
It is not a jump statement. It is used to terminate the program or directly exit from the program without following the intermediate statements. It is a library function included instdlib.h and process.h. exit is used as: exit( )
Note: break, goto, continue, return are all keywords.
Common programming errors:-
1. while statement does not include the word do. Thus it is not logical to write
while(condition) do
{
statement;
}
2. The break and continue statements affect only the innermost loops. for e.g.
for(…………) /*loop 1*/
{
for(……………..)
/*loop2*/
{
………..
………..
break;
………..
………..
}
………..
}
The break statement would cause the exit from loop 2 but not from loop 1.