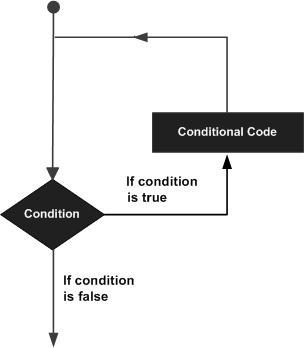
Control Statements (Looping)
Definition: Looping is a process by which we can repeat a single statement or a group of statements until some condition for termination of the loop is satisfied.
Parts of loop:-
1.Initialize (Start)
2.Condition (Stop)
3.Statement (Print)
4.Increment or Decrement (++/–)
Types of Loop:-
1. Entry control loops: These loops are those loops in which condition is checked before the execution of the statement. Thus if the condition is false in the beginning the loop will not run even once.
E.g. for loop, while loop
2. Exit control loops: These loops are those in which the condition is checked after the
execution of the statement. Thus if the condition is false in the beginning the loop will run at
least once.
E.g. do while loop
while loop: – It is the simplest of all the loops.
This loop is used as follows:
initialize;
while(condition)
{
statements;
increment or decrement;
}
While loop is an entry control loop. The condition is evaluated and if it is true the statement of the loop is executed. After the execution of statement and increment or decrement, the loop
condition is tested again. This process of repeated execution of statement, increment or
decrement and testing of condition continuous till the condition finally becomes false and the
control of the loop is transferred to the next statement.
Print the sequence 1, 2, 3, 4, 5,…………………N
int i, n;
printf(“Enter the value of N”);
scanf(“%d”, &n);
i=1;
while(i<=n)
{
printf(“%d,”,i);
i++;
}
printf(“\b”); /*to remove the comma (,) printed at the last*/
do while: – It is an exit control loop.
The loop is used as follows:
initialize;
do
{
statements;
increment or decrement
}while(condition);
Here the condition is tested after the execution of the statement and increment or decrement.
Thus in this type of loop the program proceeds to evaluate the body for the loop first and the
condition is tested after that. Thus even if the condition is false in the beginning then also the statement will be executed at least once.
Print the sequence 1, 2, 3, 4, 5,……………….N
int i ,n;
printf(“Enter the value of N ”);
scanf(“%d”, &n);
i=1;
do
{
printf(“%d,”,i);
I++;
}while(i<=n);
printf(“\b ”); /*to remove the comma(,) printed at the last*/
for loop: – for loop is also an entry loop which provides a more concise loop control structure.
The loop is used as:
for (initialize; condition; increment or decrement)
{
statements;
}
1) Here, the initialization works then the condition is evaluated if the condition is true the
execution of the statement takes first and then the increment or decrement of the variable
takes place the condition is again tested and the process goes on till the loop condition
becomes false.
2) The initialization takes place using some assignment operator. If more then one variable
is to be initialized then assignments are separated by commas.
3) After all the assignments are written a semicolon is used to separate them from
conditions. If there are more than one condition then they are separated by either logical
AND (&&) or logical OR( || ).
4) After all the conditions are given a semicolon is used before giving the increments or
decrements.
5) If there are more than one statement in the body of the loop then using the parenthesis is
compulsory.
6) A unique aspect of for loop is that one or more parts of for loop can be omitted if they are
not required. However the semicolons separating the different parts of the loop are
necessary.
7) A delay loop can also be set up by using the null statement: for(i=100;i>0;i–);
8) In this loop there is no statement thus first the variable i is initialized by 100, the
condition is tested, then the decrement takes place, again the condition is tested, i is
decreased again and it is repeated till the condition becomes false.
9) Another unique feature of the for loop is nesting that is placing one loop as a statement
for another loop. In ANSI C the nesting is allowed up to 15 levels, however some
compilers allow even more.