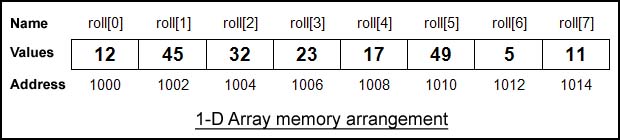
Need for an array:-
When the number of variables of same type and nature are more than it is difficult to handle them. So we need an array. Let us understand the use of array with an example:
int i,a;
for(i=1;i<=5;i++)
{
printf(“Enter the no.”);
scanf(“%d”,&a);
}
In the above program as soon as the new value is assigned to the variable a the old value is lost. Thus all the variables that we have used so far are not able to hold more than one value at a time. But sometimes we wish to store more than one value for a variable.
For example if we wish to arrange the marks of 10 students in ascending order. There are two ways for it:
(i) Use of 10 different variables so that each variable can store marks of a single student.
(ii) Use of a single variable which can store the marks of all students.
Obviously the second option looks better because it is easier to handle them. A single variable which can store more than one value at a time is called an Array.
ARRAY:
It is a collection of homogeneous data elements stored continuously under a single name.
Array declaration:-
int a[6];
here a is the name of the array, int is the data type of values which the array will store, 6is the size of array and [] are called as subscript operator. The size of array must be a constant.
The most important thing is that all the values in the array should be of the same type.
Address
100
102
104
106
108
110
Elements
10
20
30
40
50
60
Index
0
1
2
3
4
5
10,20,30,40,50 are the elements of the array. To use the elements of the array we refer to the index. The array index starts from 0 i.e. if the size of the array is 6, so the indices of the array will be from 0 to 5. In the definition we also said that the elements of the array are stored continuously,i.e. in the element at 0 index has address 100 then the next elements will have addresses 102, 104, 106, 108, 110 if the array is an integer array because an integer occupies only 2 bytes. But if it was a float array the addresses will have been 100, 104, 108, 112, 116, 120 since a float number occupies 4 bytes. If there are more than one array of the same size are to be
used in a program then another type of declaration can be used.
For example if the percentage of the students of a class is to be calculated and the marks of all the students is input in arrays. Then all the arrays should be of same size. So this type of declaration can be used:
#define SIZE 10
main( )
{
int maths[SIZE], hindi[SIZE], english[SIZE];
}
Here SIZE is a global or symbolic constant.
Array Initialize:
1. int a[5]; /*This array will contain garbage values*/
2. int a[5]={10,20,30,40,50}; /*This array will contain 10,20,30,40,50 values*/
3. int a[5] = {0,0,0,0,0}; /*This array will contain 0,0,0,0,0 values*/
4. int a[5] = {0}; /*This array will contain 0,0,0,0,0 values*/
5. int a[5] = {1}; /*This array will contain 1,0,0,0,0 values*/
6. int a[ ] = {10,20,30}; /*The size of this array will be assumed as 3*/
7. int a[3] = {10,20,30,40}; /*Too many initializes error*/
8. Static int a[5]; /*This array will contain 0,0,0,0,0 values*/
Note 1: If the size of the array is missing but the values are given then the size of the array formed will be equal to the number of initializes.
Note 2: If the number of initializes is more than the size of the array then the error of too many initializes is given.
Limitations of array:-
1. The array formed will be homogeneous. That is in an integer array only integer values can be stored, while in a float array only floating values and character array can have only characters. Thus no array can have values of two data types.
2. While declaring the array passing size of the array is compulsory, and the size must be a constant. Thus there is either shortage or wastage of memory.
3. Insertion or deletion of elements in an array will require shifting.
4. The array does not check its boundaries: In C there is no check to see if the values entered in the array are exceeding the size of the array. Data entered with the subscript exceeding the array size will be simply placed outside the array, probably on the top of the data or the program itself. This will lead to unpredictable results, to say the least, and there will be no error message to warn the programmer of going beyond the array size. In
some cases the program may hang. Thus the following program can give undesired result:
int a[10],i;
for(i=0;i<=20;i++)
a[i]=i;