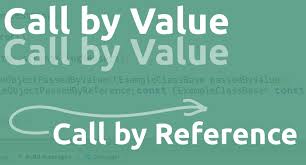
Argument Passing Mechanism:-
(i) Call by value:-
When arguments are passed by value then the copy of the actual parameters is transferred from calling function to the called function definition in formal parameters.
Now any changes made in the formal parameters in called function definition will not be reflected in actual parameters of calling function.
Like in the above function to calculate the sum of two numbers the calling statement was written as: ans = sum(a, b); Here the values of variables a, b are passed from the main function to the calling function’s definition.
In the definition variables x, y accept the values of a, b respectively. Here , the variables a, b are actual arguments while x, y will be called the formal arguments.
The scope of the actual and formal arguments is different so any change made in the formal arguments will not be seen in the actual arguments.
e.g.:
void swap(int, int);
main( )
{ int a,b;
printf(“Enter 2 numbers”);
scanf(“%d%d”,&a,&b);
swap(a,b); /*In this call statement a,b are the actual parameters*/
printf(“%d \t%d”a,b);
}
void swap(int x,int y) /*In this function definition x & y are the formal parameters*/
{
int t; t=x;x=y;y=t;
}
NOTE: In the above e.g. changes made in x, y will not be reflected in a,b.
(ii) Call by reference:-
When arguments are passed by reference then the address of the actual parameters is transferred from calling function to the called function definition in formal parameters.
Now any changes made in the formal parameters in called function definition will be reflected in actual parameters of calling function.
Sometimes it is not possible to pass the values of the variables, for example while using an array it will not be possible to pass all the values of the array using call by value.
So, another type of function calling mechanism is used call by reference where the address of the variable is passed.
Here the definition would work by reaching the particular addresses. This method is generally used for the array and pointers.
e.g.:
main( )
{
int a,b;
printf(“Enter 2 numbers”);
scanf(“%d%d”,&a,&b);
swap(&a, &b); /*In this call statement address of a, b gets transferred*/
printf(“%d \t%d”,a,b);
}
void swap(int *p1, int *p2) /*In this function definition p1 & p2 are the pointers which receive the address of a,b*/
{
int t; t=*p1; *p2; *p2=t;
}
NOTE 1: In the above e.g. changes made in p1,p2 will be reflected in a, b automatically.
NOTE 2: Arrays are also passed by reference. When we pass the name of the array then only the base address is transferred in the function definition.